In Android there is a very simple way to add a date picker to your app with just a few lines of code. In this tutorial we are going to show you how to set up the DatePicker, show the date in a TextView and calculate the age based on the users input.
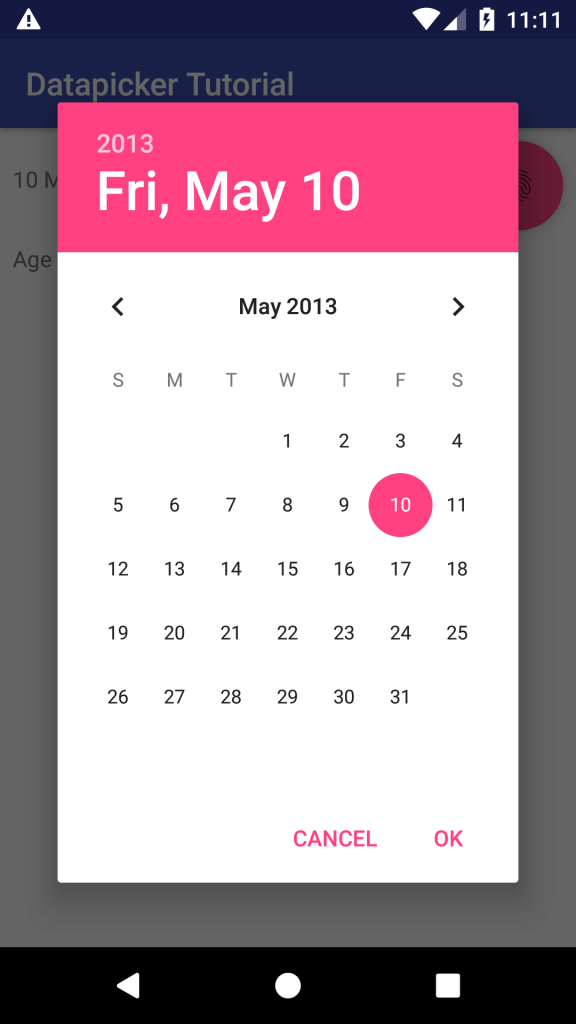
First start a new Android project with a empty Activity. Then open the activities layout file and add a textview named tvDate, another textview tvAge and a floating action button called fabDate. So your layout will look something like this
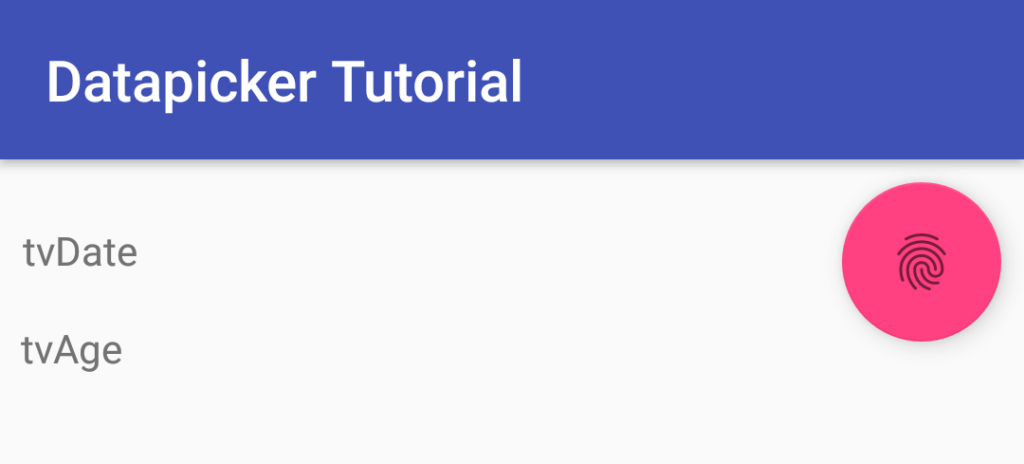
Now we go to the activities .java file and start coding. We first need to create three variables for the views in the layout. Put them in the class above the onCreate method.
FloatingActionButton fabDate;
TextView tvDate;
TextView tvAge;
Then we need to link them to the views in the layout. In the onCreate method put the following line below setContentView(…)
fabDate = findViewById(R.id.fabDate);
tvDate = findViewById(R.id.tvDate);
tvAge = findViewById(R.id.tvAge);
Now that we setup the variables for the views in the layout file we can continue with the DatePicker. When a user clicks the Floating Action Button we want a datepicker to show. We do this with the onClickListener. Put the following code below tvAge = …
fabDate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
final Calendar c = Calendar.getInstance();
int mYear = c.get(Calendar.YEAR);
int mMonth = c.get(Calendar.MONTH);
int mDay = c.get(Calendar.DAY_OF_MONTH);
DatePickerDialog dateDialog = new DatePickerDialog(view.getContext(), datePickerListener, mYear, mMonth, mDay);
dateDialog.getDatePicker().setMaxDate(new Date().getTime());
dateDialog.show();
}
});
In the code above we get an instance of the Calendar and set the date to today. The we initiate a new DatePickerDialog, set the date to the date of the Calendar we just create (today) and finally we call the .show() method to show the dialog.
So far so good but the dialog doesn’t return anything yet. To get the date that is set in the datepicker by the user we need to add a OnDateSetListener. In the code above this listener is called datePickerListener. Let’s add the listener code. This is placed beneath the onCreate method (not inside it!).
private DatePickerDialog.OnDateSetListener datePickerListener = new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker datePicker, int year, int month, int day) {
Calendar c = Calendar.getInstance();
c.set(Calendar.YEAR, year);
c.set(Calendar.MONTH, month);
c.set(Calendar.DAY_OF_MONTH, day);
String format = new SimpleDateFormat("dd MMM YYYY").format(c.getTime());
tvDate.setText(format);
tvAge.setText(Integer.toString(calculateAge(c.getTimeInMillis())));
}
};
The code above sets an instance of the Calendar to the date selected in the datepicker and then formats it to a readable date. This is then used to display the date in the tvDate textview.
In the last line we set the text in the tvAge textview to the age we calculate. To calculate the age we use a method called calculateAge. Put the following code below the datePickerListener method.
int calculateAge(long date){
Calendar dob = Calendar.getInstance();
dob.setTimeInMillis(date);
Calendar today = Calendar.getInstance();
int age = today.get(Calendar.YEAR) - dob.get(Calendar.YEAR);
if(today.get(Calendar.DAY_OF_MONTH) < dob.get(Calendar.DAY_OF_MONTH)){
age--;
}
return age;
}
The code above sets two Calendars, one with the date selected by the user and another with the current date. It then simply distracts the two dates and corrects in case the user does not had his/her birthday this year.
This is all you have to do to get a working date picker in your app.
I also have a YouTube channel with videos for some of the articles on this website. If you enjoy them, please subscribe and I will regular upload new tips and tricks.
This tutorial is also available as a video